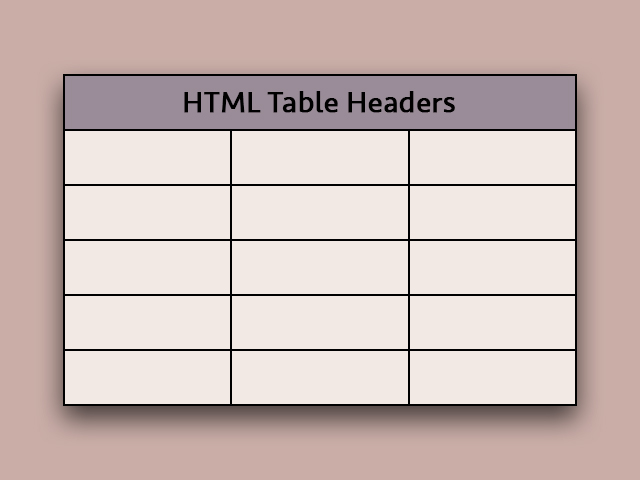
Table headers are specified with <th> tag (each th element represents a table cell).
Demo:
Name | Surname | Occupation |
---|---|---|
John | Doe | Web Developer |
Martha | Brown | Teacher |
Matt | Smith | Plumber |
Name | Occupation | |
---|---|---|
John | Doe | Web Developer |
Martha | Brown | Teacher |
Matt | Smith | Plumber |
Customer details | ||
---|---|---|
Name | Surname | |
Joe | Doe | joedoe@example.com |
Martha | Brown | brown221@example.com |
The HTML table can have headers for each column/row.
Example:
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body>
<table style="width:100%">
<tr>
<th>Name</th>
<th>Surname</th>
<th>Occupation</th>
</tr>
<tr>
<td>John</td>
<td>Doe</td>
<td>Web Developer</td>
</tr>
<tr>
<td>Matt</td>
<td>Smith</td>
<td>Plumber</td>
</tr>
<tr>
<td>Martha</td>
<td>Brown</td>
<td>Teacher</td>
</tr>
</table>
</body>
</html>
Output:
Name | Surname | Occupation |
---|---|---|
John | Doe | Web Developer |
Martha | Brown | Teacher |
Matt | Smith | Plumber |
You can create a header that spans over two columns (or more).
Example1:
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body>
<table style="width:100%">
<tr>
<th colspan="2">Name</th>
<th>Occupation</th>
</tr>
<tr>
<td>John</td>
<td>Doe</td>
<td>Web Developer</td>
</tr>
<tr>
<td>Martha</td>
<td>Brown</td>
<td>Teacher</td>
</tr>
<tr>
<td>Matt</td>
<td>Smith</td>
<td>Plumber</td>
</tr>
</table>
</body>
</html>
Output:
Name | Occupation | |
---|---|---|
John | Doe | Web Developer |
Martha | Brown | Teacher |
Matt | Smith | Plumber |
Example2:
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
th {
text-align: center;}
</style>
</head>
<body>
<table style="width:100%">
<tr>
<th colspan="3">Customer details</th>
</tr>
<tr>
<th>Name</th>
<th>Surname</th>
<th>e-mail</th>
</tr>
<tr>
<td>Joe</td>
<td>Doe</td>
<td>joedoe@example.com</td>
</tr>
<tr>
<td>Martha</td>
<td>Brown</td>
<td>brown221@example.com</td>
</tr>
</table>
</body>
</html>
Output:
Customer details | ||
---|---|---|
Name | Surname | |
Joe | Doe | joedoe@example.com |
Martha | Brown | brown221@example.com |
Enjoy coding!
Read also: